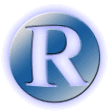
How to get the "Ordinal" for any number in javaScript (1st, 2nd, 3rd, 4th)
I came across a need to display numbers with "ordinals" in one of our pages at Foxsports.com.
For those of you who don't know what an ordinal number is, here is the short definition: the "st", "nd", "rd" after a "number". example: 1st, 2nd, 3rd, 4th, etc.
I didn't see any scripts out there that did the mathematics for this properly, so I created one that works pretty well. Check it out!
What it does:
- It will take whatever number you pass it and return the correct ordinal for that particular number.
- ex: if you pass in "3", it will return "3rd"... or pass in "2011", it will return "2001th".
Options:
- subScript: // values: sub or sup... this will return the number with the ordinal surrounded by an HTML sub script tag or super script tag
How to call it:
ordinal ( 15 , { sScript: "sup" } );
The result will be: 15th.
** Click here to DOWNLOAD the script **
Here is the code:
/* BEGIN: [Ordinal Number Format v.1 :: Author: Addam Driver :: Date: 10/5/2008 ] ** Disclaimer: Use at your own risk, copyright Addam Driver 2008 ** Example on calling this function: ** ordinal(this, {subScript: true}); ** The Ordinal script will append the proper ordinal to any number like: 1st, 2nd, 33rd, etc. ** If you pass the "subScript:true" option, then it will use the html subscript for the new ordinal */ function ordinal(num, options){ var options = options || {}; // setup the options var sScript = options.sScript; // get subscript if needed var mod1 = num%100; // get the divided "remainder" of 100 var mod2 = num%10; // get the divided "remainder" of 10 var ord; // ste the ordinal variable if((mod1-mod2) == 10){ // capture 10 ord = "th"; // set the oridnal to th as in: 10th }else{// for everything else switch(mod2){ // check the remainder of the 10th place case 1: // if 1 as in 1st ord = "st"; // set the ordinal break; case 2: // if 2 as in 2nd ord = "nd";// set the ordinal break; case 3: // if 3 as in 3rd ord = "rd";// set the ordinal break; default: // for everything else ord = "th";// set the ordinal break; } } switch(sScript){ case "sub": return num+"<sub>"+ord+"<\/sub>"; // put the ordinal in the HTML sub script break; case "sup": return num+"<sup>"+ord+"<\/sup>"; // put the ordinal in the HTML super script break; default: return num+ord; break; } }
I hope this helps someone out there! Cheers!