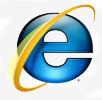
JavaScript 5 Star Rating System
I was asked to create a 5 star rating system for a few shows on NBC.com. So I created the following code. This is just the scaled down version but it works pretty well. You will need to add in extra code to send the results somewhere using Ajax or something like that. Try it out!
The idea here was to make this thing as simple as possible to add as many stars as you want without having to write out some long crazy code to make this happen. I'm sure I could simplify this a little more, I'll work on that later. For now, let's get into implementation of the stars.
The first part of the system is the HTML:
<span id="ratingSaved">Rating Saved!</span>
The first span tag is for the "Status" or what the stars/images represent. The other one is the information that will be displayed .
NEXT! The rating stars themselves:
<a onclick="rateIt(this)" id="_1" title="ehh..." onmouseover="rating(this)" onmouseout="off(this)"></a>
<a onclick="rateIt(this)" id="_2" title="Not Bad" onmouseover="rating(this)" onmouseout="off(this)"></a>
<a onclick="rateIt(this)" id="_3" title="Pretty Good" onmouseover="rating(this)" onmouseout="off(this)"></a>
</div>
If you want to add more stars, just simply add another anchor to the list and increase it's ID to keep track of it's "rating"
NEXT! Let's add some style:
#rateStatus{float:left; clear:both; width:100%; height:20px;}
#rateMe{float:left; clear:both; width:100%; height:auto; padding:0px; margin:0px;}
#rateMe li{float:left;list-style:none;}
#rateMe li a:hover,
#rateMe .on{background:url(star_on.gif) no-repeat;}
#rateMe a{float:left;background:url(star_off.gif) no-repeat;width:12px; height:12px;}
#ratingSaved{display:none;}
.saved{color:red; }
</style>
To change the "Stars" to whatever image you want to use, change the background "url" image location in the "#rateMe .on" and "#rateMe a" styles.
NEXT! Include the script and your are done!
This script can be added anywhere on the page. You can DOWNLOAD IT HERE.
Inside the script you will see a function at the bottom that say's "sendRate()". That is where you can put any random javascript, ajax, form submission etc. That will actually do something with the rating data once the user selects a rating.
Here is a working version: